Generics in C#.NET are a powerful feature that allows you to create reusable code components that can work with different data types. They provide a way to define classes, structures, methods, and interfaces that can operate on various types without sacrificing type safety. Here's a detailed explanation of generics in C#.NET:
In traditional programming, you often need to write separate code for different data types. For example, if you want to create a collection class that stores integers, you would need to create a specific implementation for integers. If you later want to use the same collection class for strings, you would need to create a separate implementation for strings. This approach leads to code duplication and can be tedious to maintain.
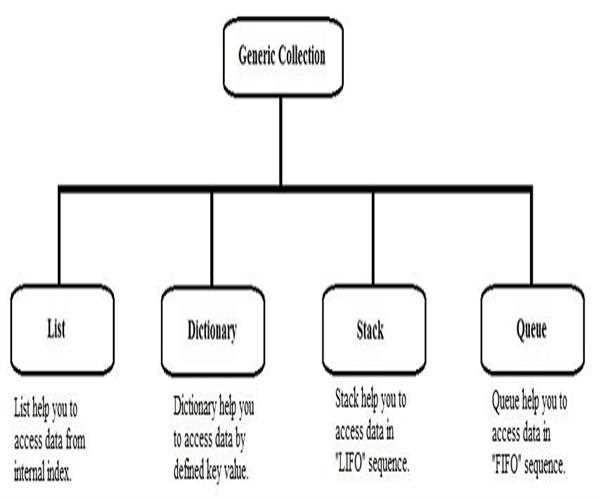
Generics solve this problem by allowing you to create classes and methods that can work with multiple types. Rather than specifying the exact data type, you can define placeholders called type parameters. These type parameters can be replaced with actual types when using the generic component.
For example, you can define a generic class called List<T>, where T is a type parameter representing the type of elements stored in the list. When you create an instance of List<int>, T is replaced with int, creating a specialized list that can only store integers. Similarly, List<string> creates a list that can store strings. The same generic class can be reused for any other type.
Generics provide several benefits:
Reusability: With generics, you can write code that can be used with different data types, promoting code reuse and reducing redundancy. This leads to cleaner and more maintainable code.
Type Safety: Generics ensure type safety at compile-time. By specifying the type parameter, the compiler enforces type constraints, preventing invalid type operations. This eliminates runtime type errors and enhances code reliability.
Performance: Generics offer performance benefits by avoiding unnecessary type conversions or boxing/unboxing operations. Since the code is generated for each specific type at compile-time, it eliminates the need for runtime type checks.
Code Flexibility: Generics provide flexibility by allowing you to create generic classes, methods, interfaces, and delegates. This enables you to create components that can work with different types of data, making your code more adaptable and versatile.
Collections and Algorithms: Generics are extensively used in collections and algorithms provided by the .NET framework. The List<T>, Dictionary<TKey, TValue>, and IEnumerable<T> are some examples of generic collection classes. These generic collections improve performance and type safety while working with data.
Custom Data Structures: Generics allow you to create custom data structures tailored to specific types. You can create generic stacks, queues, linked lists, and more, providing type-safe and efficient data storage and manipulation.
In conclusion, generics in C#.NET enable you to create reusable code components that can work with different types, promoting code reuse, type safety, and performance. By using type parameters, you can define generic classes, methods, and interfaces, allowing for flexibility and efficiency in working with various data types. Generics play a significant role in the .NET framework and are widely used in collections, algorithms, and custom data structures.