Constructors are special functions used to create objects in object-oriented programming. They are typically used to initialize the object's properties and provide a way to instantiate an object of a class. A constructor is a special type of method that is used to initialize an object when it is created. It is called when an object of a class is created and can be used to set initial values, allocate memory or perform any other kind of initialization that the object may need.
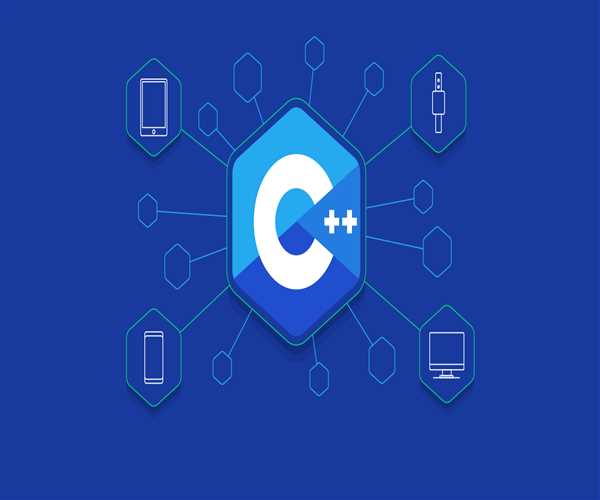
Constructors are typically declared with the same name as the class they belong to and they do not have a return type. Constructors can also be overloaded, meaning that multiple constructors can exist within a single class, differentiated by their parameters. Overloading constructors can be used to create objects with different initial data.
The basic syntax of a constructor is as follows:
class ClassName
{
ClassName()
{
// Code to execute during object creation
}
}
When creating an object of a class, the constructor is called automatically. This means that any code placed inside the constructor will be executed when the object is created. Constructors are typically used to initialize the data members of an object, which are the variables that are declared inside the class. This can be done by assigning values to them directly or by calling other methods that set the initial values.
For example, a constructor for a class that represents an employee object might look like this:
class Employee
{
String name;
int age;
double salary;
Employee()
{
name = "John Doe";
age = 25;
salary = 30000.00;
}
}
When an object of the Employee class is created, the constructor is called automatically and it sets the initial values for the name, age, and salary variables.
Constructors can also be used to perform other tasks, such as allocating memory for data structures or setting up connections to databases. This allows the object to be ready to use without any additional setup. For example, a constructor for a class that represents a database connection might look like this:
class DatabaseConnection
{
Connection conn;
DatabaseConnection()
{
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/database", "user", "password");
}
}
In this example, the constructor is used to set up a connection to a database. When an object of the DatabaseConnection class is created, the constructor is called automatically and it sets up the connection to the database.
In summary, constructors are special functions used to create objects of a class in object-oriented programming. They are typically used to initialize the object's properties and provide a way to instantiate an object of a class. Constructors can also be used to perform other tasks, such as allocating memory or setting up connections to databases, which allows the object to be ready to use without any additional setup.