In the Call by Value method, the values of the actual parameters are copied into the formal parameters of the function. In other words, 'in this, the value of the variable is used in the function call.' In the Call by value method, we cannot change the value of the actual parameter by using formal parameters. In this, the actual and formal parameters are stored in different memory locations. The actual parameter is an argument that is used in a function call while the formal parameter is an argument that is used in the function definition.
In Call by reference, the address of an argument is copied into the formal parameters. In other words, 'in this, the address of the variable is passed to the function call as the actual parameter.' In this, if we change the value of the formal parameter, then the value of the actual parameter will also change. In call-by-reference, both the actual and formal parameters are stored in the same memory location.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ThreadDemo
{
class CallByValueAndReferance
{
public CallByValueAndReferance()
{
}
// call by value function definition
public void CallByValue(int actual)
{
Console.WriteLine($'Before Changing Actual Value : {actual}');
actual += 100;
Console.WriteLine($'After Changing Actual Value : {actual}');
}
// call by reference function definition
public void CallByReferance(ref int actual)
{
Console.WriteLine($'Before Changing Actual Value : {actual}');
actual += 100;
Console.WriteLine($'After Changing Actual Value : {actual}');
}
public static void Main(string[] args)
{
CallByValueAndReferance callBy = new CallByValueAndReferance();
int val = 50;
// call by value function
callBy.CallByValue(val);
Console.WriteLine('In main() call by value ' + val);
// call by reference function
callBy.CallByReferance(ref val);
Console.WriteLine('In main() call by ref ' + val);
}
}
}
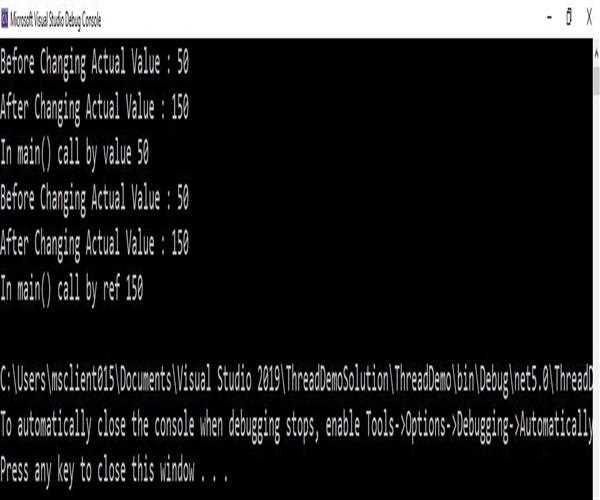