Conditional statements are those statements, which execute one or more specified statements only if a specific condition is true. The various conditional statements supported by C# are as follows:
if statement
Using this statement, we can program one or more statements to be executed or not be executed on the basis of any condition. The syntax of this statement is as follows:
if(condition)
{
// Statements to be executed when the condition true
}
According to this syntax, if Specified Condition or Expression between Parenthesis returns a true Boolean value, then Specified Statements between Curly Braces are executed, otherwise C# Program Control does not execute any Statement Specified between Curly Braces.
if … else statement
We usually use this statement when we have such a group of one or more statements of two types, some of which have to be executed when some condition is true, while some of the execution conditions to be false. The syntax of this statement is as follows:
if(condition)
{
// Statements to be executed when condition true
}
else
{
// Statements to be executed when condition false
}
According to this syntax, if the specified condition or expression between parenthesis returns a true Boolean value, then the specified statements are executed in the if statement block, otherwise C# Program Control executes the specified statements in the else statement block.
else if … Statement
We generally use this statement when we have a group of more than two types of one or more statements, which have to be executed only if there is a match of a particular condition. The syntax of this statement is as follows:
if(condition)
{
// Statements to be executed when condition true
}
else if(condition)
{
// Statements to be executed when condition true
}
else if(condition)
{
// Statements to be executed when condition true
}
else
{
// Statements to be executed when all other conditions false
}
According to this Syntax, if Specified Condition or Expression between Parenthesis returns true Boolean Value, then Specified Statements are executed in the If Statement Block, otherwise C# Program Control will test the Specified Condition or Expression between the next else If Parenthesis And in case of true, executes the specified statements in this else if statement block, otherwise tests the specified condition or expression between the next else if parenthesis and this process continue till all the if-else statements Tests are not done. Whereas if no if or else if statement block is executed, then by default the else statement block gets executed.
Branching Control Statements or switch statement in C#
C# also has a switch statement defined like C/C++ and Java, which is an Unconditional Branching Statement. That is, when we use this statement, we get the facility to execute one or more statements, not on the basis of any kind of condition, but on the basis of the result generated from an expression.
switch(expression)
{
case value1:
//Statements to be executed if returned value of expression matches with value1
break;
case value2:
//Statements to be executed if returned value of expression matches with value2
break;
case value3:
//Statements to be executed if returned value of expression matches with value3
break;
default:
//Statements to be executed if returned value of expression matches with value1
break;
}
In this statement, if the value generated from the specified expression between the switch parenthesis matches with case value1, then the statement block of case value1 is executed. If the case value2 matches, then the statement block of case value2 is executed. If the case value3 matches, then the statement block of case value3 is executed. Whereas if it does not match with any case, then by default the Statement Block of the default case gets executed.
Example
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ThreadDemo
{
class SelectionStatement
{
public static void Main(string[] args)
{
SelectionStatement selection = new SelectionStatement();
Console.WriteLine('Student Grade : '+selection.FindGrade(45, 85));
selection.FindVovel('Mindstick Software pvt ltd');
}
public char FindGrade(params double[] numbers)
{
double result = 0;
foreach (double item in numbers)
{
result += item;
}
result = result / (numbers.Length);
if (result > 80 && result <= 100)
return 'A';
else if (result > 60 && result <= 80)
return 'B';
else if (result > 40 && result <= 60)
return 'C';
else
return 'F';
}
public void FindVovel (string str)
{
foreach (char item in str.ToCharArray())
{
string result = this.checkVovel(item) ? ' Vovel.' : ' Consonant.';
Console.WriteLine($'{item} is {result}');
}
}
private bool checkVovel(char ch)
{
switch (ch = Char.IsUpper(ch) ? char.ToLower(ch):ch)
{
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
return true;
default:
return false;
}
}
}
}
Output:
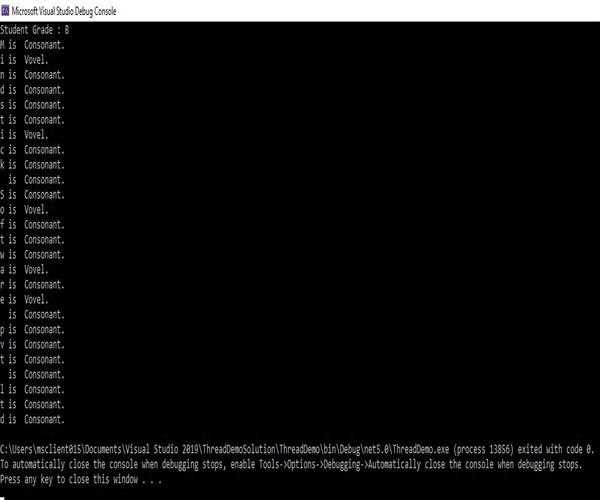