Getting started first we know about What is summernote? it is an editor that uses an open source library for posting any text or image. It is a combination of different language like HTML, CSS, javascript, jQuery, Bootstrap..etc.
Here we demonstrate the simple example for adding the summernote editor in our code project.
Add this code to your dependencies
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
..........................
........................
dependencies {
implementation 'com.android.support:appcompat-v7:27.1.1'
implementation 'com.android.support:support-v4:26.1.0'
implementation 'com.github.bumptech.glide:glide:4.0.0'
compile 'in.nashapp.androidsummernote:androidsummernote:1.0.5'
}
Add some code in Manifest (For adding images from Device)
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
Retrive HTML Data from summernote
String html_data = summernote.getText();
Set HTML Data
summernote.setText("<h2><i>Hello World.</i><h2><br><h3> Summernote Example</h3>");
Activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.msclient009.summernoteexample.MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/tex1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:layout_marginStart="50dp"
android:layout_marginTop="20dp"
android:text="Summernote Example"
android:textColor="#f00"
android:textSize="22dp"
tools:ignore="InvalidId" />
<TextView
android:id="@+id/tex"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:layout_marginStart="50dp"
android:layout_marginTop="20dp"
android:text="Scrollable Summernote"
android:textColor="#f00"
android:textSize="22dp"
tools:ignore="InvalidId" />
<in.nashapp.androidsummernote.Summernote
android:id="@+id/summernote"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_marginEnd="5dp"
android:layout_marginStart="5dp"
android:layout_marginTop="5dp"
android:overScrollMode="ifContentScrolls"
android:scrollbarAlwaysDrawVerticalTrack="true"
android:scrollbarSize="20dp"
android:scrollbarStyle="insideInset"
android:scrollbars="vertical"
android:verticalScrollbarPosition="right" />
</LinearLayout>
</ScrollView>
MainActivity.java
package com.example.msclient009.summernoteexample;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.MotionEvent;
import android.view.View;
import in.nashapp.androidsummernote.Summernote;
public class MainActivity extends AppCompatActivity implements View.OnTouchListener {
Summernote summernote;
@SuppressLint("ClickableViewAccessibility")
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
summernote = (Summernote) findViewById(R.id.summernote);
summernote.setRequestCodeforFilepicker(5);
summernote.setText("<h2>Hello World.<h2><br><h3> I'am Summernote</h3>");
summernote.setOnTouchListener(MainActivity.this);
}
@Override
public boolean onTouch(View view, MotionEvent motionEvent) {
if (summernote.hasFocus()) {
view.getParent().requestDisallowInterceptTouchEvent(true);
switch (motionEvent.getAction() & MotionEvent.ACTION_MASK){
case MotionEvent.ACTION_SCROLL:
view.getParent().requestDisallowInterceptTouchEvent(false);
return true;
}
}
return false;
}
}
Output :
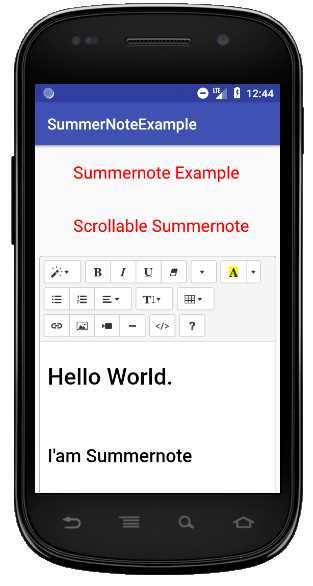
"Thanks for Reading"