- Arithmetic Operators
- Assignment Operators
- Increment and Decrement Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Ternary Operators
Arithmetic Operators
In C#, Arithmetic operators are used to performing basic arithmetic operations like addition, subtraction, division, etc.
Relational Operators
In C#, relational operators are used to checking the relation between two operators, such that we can determine whether two operand values are equal or not, depending on our requirements.
Logical Operators
In C#, the logical operators AND, and NOT are used to perform logical operations between two operators based on our requirements, and the operators must contain only boolean values otherwise the logical operator will show an error.
Bitwise Operators
Bitwise operator is used to performing bit manipulation operations, in C#, Bitwise operator operates on bits and it is useful for performing a bit on bit operations like bitwise AND bitwise or bitwise exclusive or (^), etc. boolean and Level operations on integer data.
Assignment Operators
In C#, the assignment operator is used to assigning the new value to the operand.
Ternary Operator
The ternary operator works on three operators. It is a shorthand for the if-then-else statement. Ternary operator can be used as follows.
Example
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ThreadDemo
{
class OperatorDemo
{
public void ArithamaticOperator(params int[] val)
{
int sumResult = 0;
int subResult = 0;
foreach (int item in val)
{
sumResult += item;
subResult -= item;
}
Console.WriteLine($'Sum : {sumResult} and Sub : {subResult}');
}
public void GretestInThreeNumber(int first,int second, int thierd)
{
if( ((first + second + thierd) / 3) == first)
{
Console.WriteLine('All values are same ...');
}else if(first>second && first>thierd)
{
Console.WriteLine($'First is grater {first}');
}else if (second > thierd)
{
Console.WriteLine($'Second is grater {second}');
}
else
{
Console.WriteLine($'Thierd is grater {thierd}');
}
}
public void PrintData(int length)
{
for (int i = 1; i <= length; i++)
{
string space = i <= length-1 ? ',' : '\n';
Console.Write(i + '' + space);
}
}
public static void Main(string[] args)
{
OperatorDemo ope = new OperatorDemo();
ope.ArithamaticOperator(5, 8, 4, 9, 6, 2, 3, 3, 40);
ope.GretestInThreeNumber(10, 15, 10);
ope.PrintData(20);
}
}
}
Output
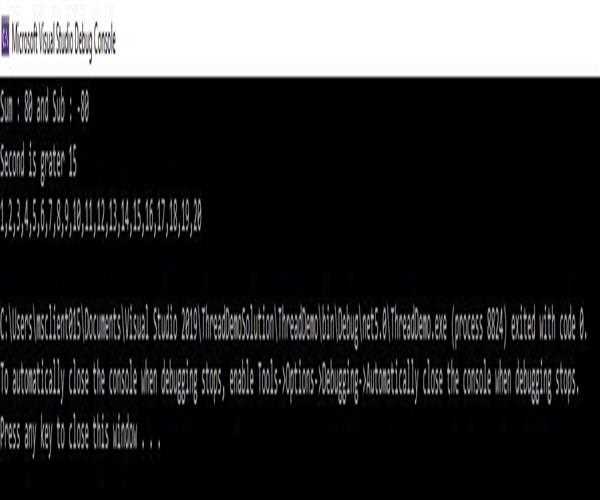