SOLID is a set of five design principles for software development that were introduced by Robert C. Martin (also known as Uncle Bob). The SOLID principles help developers to create software that is easy to understand, maintain, and extend. The principles can be applied to any programming language, including C#. In this section, we will discuss each of the five SOLID principles in detail.
Single Responsibility Principle (SRP) The SRP states that a class should have only one reason to change. This means that a class should have only one responsibility and should not be responsible for multiple things. This helps to keep the code organized and makes it easier to maintain and extend.
Open/Closed Principle (OCP) The OCP states that a class should be open for extension but closed for modification. This means that you should be able to add new functionality to a class without changing its existing code. This helps to keep the code stable and reduces the risk of introducing bugs.
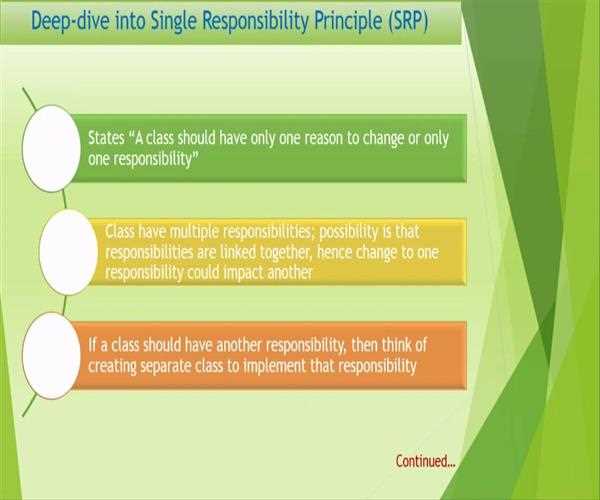
Liskov Substitution Principle (LSP) The LSP states that a subclass should be able to be substituted for its parent class without causing any errors. This means that the behavior of the subclass should not be different from its parent class. This helps to maintain consistency and reduces the risk of errors in the code.
Interface Segregation Principle (ISP) The ISP states that a class should not be forced to implement interfaces that it does not use. This means that an interface should be specific to the needs of the class and should not include unnecessary methods. This helps to keep the code clean and makes it easier to understand and maintain.
Dependency Inversion Principle (DIP) The DIP states that high-level modules should not depend on low-level modules. Instead, both should depend on abstractions. This means that the code should be designed in such a way that the high-level modules can work independently of the low-level modules. This helps to make the code more flexible and easier to maintain.
In summary, the SOLID principles are a set of guidelines that help developers to create software that is easy to understand, maintain, and extend. By following these principles, developers can write code that is more organized, stable, and flexible. In C#, the SOLID principles can be applied to classes, interfaces, and modules to create clean and maintainable code.