Broadcast Receivers in Android:
Broadcast Receiver is used to broadcast a messages from one application to another applications or our own system itself. For some time this message is called intents or event. And when any event occur then broadcast are sent.
Another example, the Android system sends broadcasts when any types of events occur in your system, such as when the system starting the boots up and the device connect for charging. In this situation, apps can also send custom broadcasts to notify other apps are might be downloaded or uploaded.
When a broadcast is send to any system, the system automatically routes broadcasts to your apps that have subscribed to receive that particular type of broadcast. Generally speaking, broadcasts receiver can be used as a messaging system across apps and outside of the normal user flow. It is used to perform jobs in the background and can be contribute to a slow system performance.
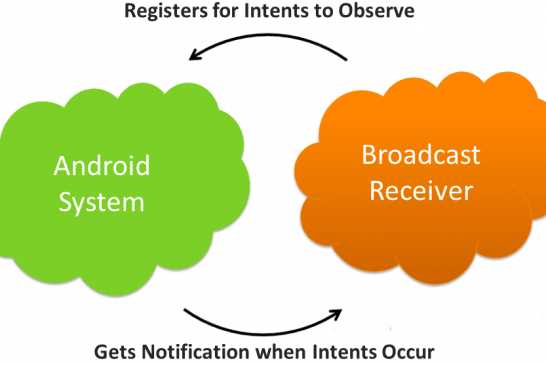
If you want to set up a Broadcast Receiver in your android app you need to do the following two things.
1. Creating a BroadcastReceiver
2. Registering a BroadcastRecever
Creating a broadcast receiver in Android:
public class MyBroadcastReceiver extends BroadcastReceiver {
public MyBroadcastReceiver () {
}
@Override
public void onReceive(Context context, Intent intent) {
Toast.makeText(context, "Action: " + intent.getAction(), Toast.LENGTH_SHORT).show();
}
}
Note: When any event occurs then onReceiver() method is first called for registered the Broadcast Receivers.
Registering the Broadcast Receiver in Android:
A BroadcastReceiver can be registered in mainly two ways.
1. Firstly you can define the code in AndroidManifest.xml file is as follows-
<receiver android:name=".ConnectionReceiver" >
<intent-filter>
<action android:name="android.net.conn.CONNECTIVITY_CHANGE" />
</intent-filter>
</receiver>
2. By Using Code (programmatically).
IntentFilter filter = new IntentFilter();
intentFilter.addAction(getPackageName()+"android.net.conn.CONNECTIVITY_CHANGE");
MyReceiver myReceiver = new MyReceiver();
registerReceiver(myReceiver, filter);
For unregister a broadcast receiver you can call onStop() or onPause() method to your activity.
@Override
protected void onPause() {
unregisterReceiver(myReceiver);
super.onPause();
}
Sending the broadcast intent from an activity
Intent intent = new Intent ();
intent.setAction("com.mindstick.CUSTOM_INTENT");
sendBroadcast(intent);