AsyncTask is an abstract class that provided by android to perform
lot of operation or huge amount of task in the background and keep the UI thread more light and create an application more responsive.
In android application, each android app runs on a single thread when it is compiled. And single thread take a longer time to fetching the response to the application and make the application non responsive. For avoiding this concept android provided AsyncTask abstract class that perform heavy tasks in the background and result go back to the UI thread. If you are using AsyncTask abstract class in our android application keeps the UI thread more responsive at all times when you run your application.
There are some following methods that are used in AsyncTask class are as follows-
1. onPreExecute() : This method executes before background processing starts.
2. doInBackground() : This method contain those code that perform operation on the background. And send the result multiple times to the UI thread by calling publishProgress() methods. After completing whole background processing you can use return statements.
3. onPostExecute() : This method is called after doInBackground() method complete the processing of task. And after that competing the task doInBackground() method send the result on this method.
4. onProgressUpdate() : When receiving the updates from doInBackground() method after that this method is called. And this methods is used to update the UI thread on your Application.
AsyncTask can take three arguments-
- Params: It defines the type of parameters that you send for execution.
- Progress : Which type of progress running for background computation.
- Result: It define the type of result for the background computation.
AsyncTask Example :
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.msclient009.asynctaskexample.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Enter Sleeping time in second : " app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintLeft_toLeftOf="parent" android:textSize="25dp" android:textStyle="bold" android:textColor="#f00" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" app:layout_constraintVertical_bias="0.032" android:id="@+id/textView1" /> <EditText android:id="@+id/editText1" android:layout_width="match_parent" android:layout_height="60dp" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_below="@+id/textView1" android:layout_marginTop="20dp" android:background="@android:drawable/editbobackground" android:inputType="number" tools:layout_editor_absoluteX="93dp" tools:layout_editor_absoluteY="54dp" /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/editText1" android:layout_centerHorizontal="true" android:layout_marginTop="27dp" android:text="Run Async task" android:textSize="25dp" android:textStyle="bold" tools:layout_editor_absoluteX="66dp" tools:layout_editor_absoluteY="125dp" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:textColor="#00f" android:textSize="18dp" /> </RelativeLayout>
MainActivity.java
package com.example.msclient009.asynctaskexample; import android.app.ProgressDialog; import android.os.AsyncTask; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; public class MainActivity extends AppCompatActivity { private Button button; private EditText time; private TextView result; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); time = (EditText) findViewById(R.id.editText1); button = (Button) findViewById(R.id.button1); result = (TextView) findViewById(R.id.textView2); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { AsyncTaskRunner runner = new AsyncTaskRunner(); String sleepTime = time.getText().toString(); runner.execute(sleepTime); } }); } private class AsyncTaskRunner extends AsyncTask<String, String, String> { private String resp; ProgressDialog progressDialog; @Override protected void onPreExecute() { progressDialog = ProgressDialog.show(MainActivity.this, "ProgressDialog", "Wait for "+time.getText().toString()+ " seconds"); } @Override protected String doInBackground(String... params) { publishProgress("Sleeping..."); // Calls onProgressUpdate() try { int time = Integer.parseInt(params[0])*1000; Thread.sleep(time); resp = "Slept for " + params[0] + " seconds"; } catch (InterruptedException e) { e.printStackTrace(); resp = e.getMessage(); } catch (Exception e) { e.printStackTrace(); resp = e.getMessage(); } return resp; } @Override protected void onPostExecute(String result1) { // execution of result of Long time consuming operation progressDialog.dismiss(); result.setText(result1); }
@Override protected void onProgressUpdate(String... text) { result.setText(text[0]); }
} }
output :
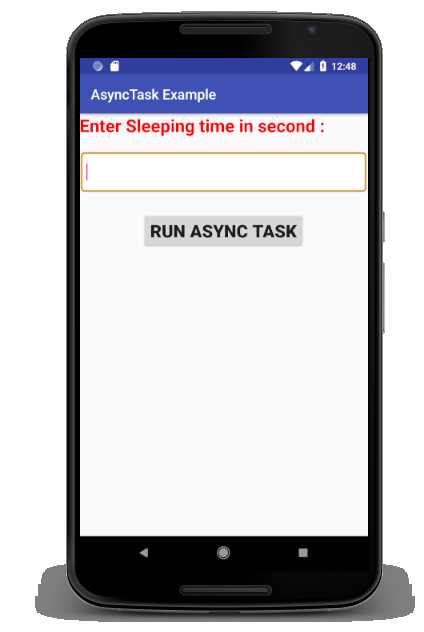
After clicking on Button.
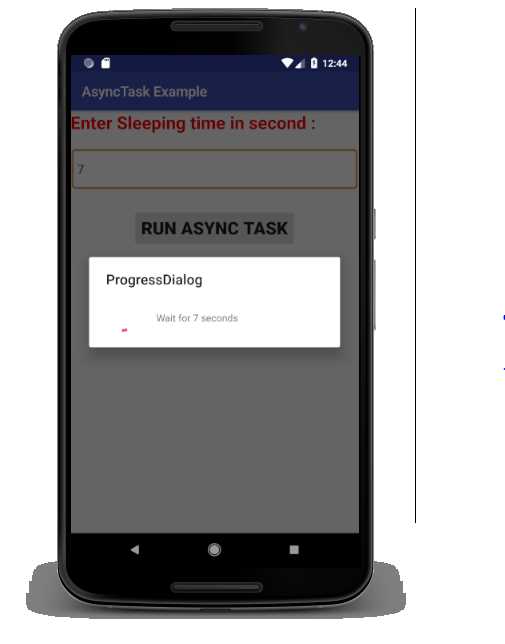
Final Output
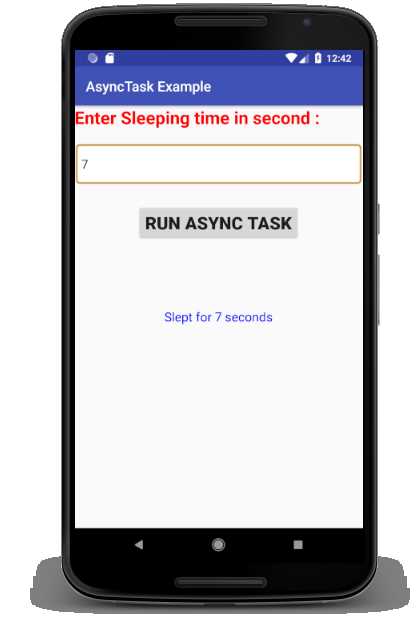