There are two ways to send data from one activity to another activity in android.
1. By Using Intent
2. By using Global Variable
Here we are using Intent, It is also used to send data from one activity to another activity using putExtra() function. The data is passed in key value pair form. The value can be any types like int, float, long, string, etc.
Sending Data
Intent intent=new Intent(context,AnotherClassName); intent.putExtra(key, value); startActivity(intent);
Receiving Data
If the data is sent string type then it can be receive in following ways.
Intent intent=getIntent(); String str=intent.getStringExra(Key);
There are several other methods like getIntExtra() for int, getFloatExtra() for float, etc to fetch other types of data.
Example
Below is the example, a name is passed from first activity to second when a button is pressed.
activity_first_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.example.msclient009.opennewactivity.FirstMainActivity"> <TextView android:id="@+id/textView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignLeft="@+id/editText" android:layout_alignParentTop="true" android:layout_alignStart="@+id/editText" android:layout_marginTop="49dp" android:text="This is First Activity" android:textColor="#f00" android:textSize="20dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> <EditText android:id="@+id/editText" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" android:layout_marginTop="79dp" android:hint="Enter Your Name...." /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_below="@+id/editText" android:layout_marginLeft="25dp" android:layout_marginStart="25dp" android:layout_marginTop="63dp" android:text="Click Here to open new Activity and Send Your Name" /> </RelativeLayout>
FirstMainActivity.java
package com.example.msclient009.opennewactivity; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; public class FirstMainActivity extends AppCompatActivity { EditText editText; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_first_main); Button button=(Button)findViewById(R.id.button1); editText=(EditText)findViewById(R.id.editText);
button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { String str=editText.getText().toString(); Intent intent=new Intent(FirstMainActivity.this, NewMainActivity.class); intent.putExtra("Name", str); startActivity(intent); } }); } }
activity_second_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:layout_marginTop="110dp" android:text="This is Second Activity" android:textColor="#00f" android:textSize="30dp" /> <TextView android:id="@+id/textView2" android:layout_width="200dp" android:layout_height="wrap_content" android:layout_alignEnd="@+id/textView1" android:layout_alignRight="@+id/textView1" android:layout_below="@+id/textView1" android:layout_marginEnd="46dp" android:layout_marginRight="46dp" android:textColor="#fff" android:layout_marginTop="60dp" android:background="#f00" android:text="Arti mishra" android:textSize="30dp" /> </RelativeLayout>
FirstMainActivity.java
package com.example.msclient009.opennewactivity; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.widget.TextView; public class FirstMainActivity extends Activity { TextView textView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_second_main); textView=(TextView)findViewById(R.id.textView2); Intent intent=getIntent(); String str = intent.getStringExtra("Name"); textView.setText(str); } }
output
:
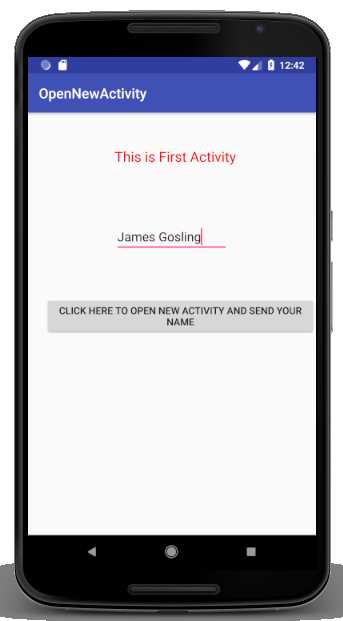
After Clicking on the button
:
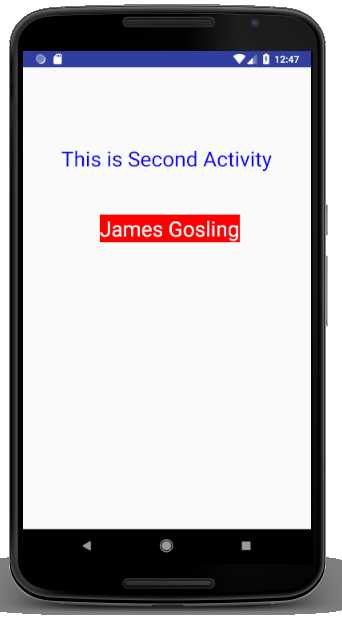